How to extract C/C++ hard-coded strings from source code to resources
Lingobit Extractor allows you to extract string constants from C and C++ source code to Window Resource Script files (.RC). It finds all strings constants and allows you to replaces them with resource loading macro. For example, code
printf(L"Hello, world!");
will be automatically replaced with
printf(_LS(IDS_STRING1));
where _LS is a function that loads string from resources, IDS_STRING1 is an automatically generated ID for string that were placed into resource script file.
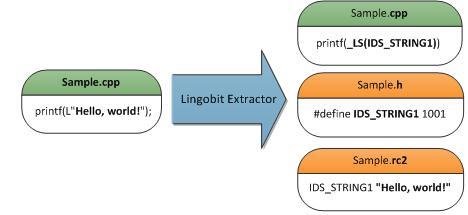
All extracted strings are stored into separate resource script file that should be manually included into the main resouce file of your application.
Setting up a project
Create a project using File\New Project menu. After it you can add files and folders with source code using Project menu. Let’s test how it works. We’ll add CPPSampleAPP folder into our project. It is distributed with Lingobit Extractor and should be stored at My Documents\Lingobit Extractor 1.0\Samples\Before\.
After you selected a folder, parse settings dialog will be shown. Here you can associate file masks with parsers. You can move items in list to set mask priority. Lines at the top will be applied first. We decided to ignore stdafx.h and stdafx.cpp files and moved this rules to the top of the list.
After you added a folder you’ll see it at the Sources panel. If you select file in Sources navigation tree the preview window will be displayed. It will highlight all hardcoded strings that could be extracted.
Creating a resource
Before extracting hardcoded strings you need to create resource file for them. Click Project\Add Resource. You need to create new separate file or select file that was created and filled with Lingobit Extractor, because the tool works only with string resources and doesn’t support files created with other IDEs. .
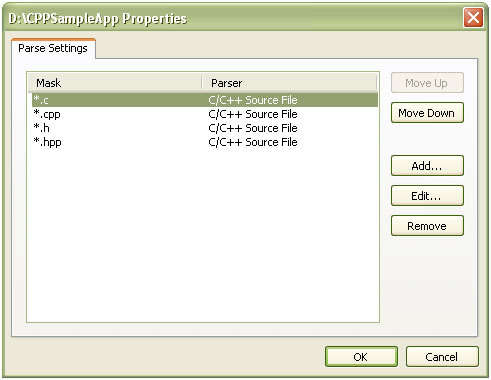
After selecting a file you can configure resource file options. In addition to name and location you can specify identifier ranges, header file and templates that can be used when extracting strings.
When specifying custom template you can use the following variables:
$(ID) – name of string identifier in resource file. It is equal to $(NUM_ID) if you selected not to create header file.
$(NUM_ID) – numeric resource identifier
$(SRC_STR) – value of hardcoded string (without quotes, brakes, and etc.)
$(SRC_TEXT) – whole string that is replaced with quotes, brakes and modifiers. For example: _T(“Hello World!”)
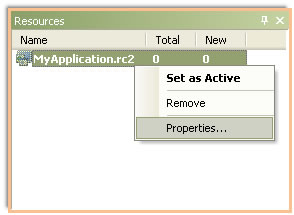
After you created resource file you can change its settings using context menu
Including extracted strings into C++ project and main resources
After you created resource file for Extractor you need to include it into you master resource file. You can do it from Microsoft Visual Studio IDE. Go to Resource View and select Resource Includes in context menu. After it type your resource file name into Compile-time directives section. You can see modified resource file in the folder My Documents\Lingobit Extractor 1.0\Samples\After\.
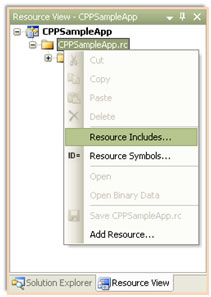 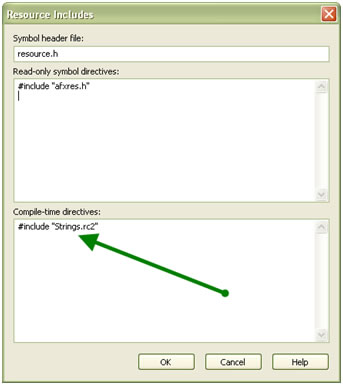
Strings.h и Strings.rc2 – header and resource files created with Lingobit Extractor
ResString.h –contains function _LS( id ) that load string from resources. This file was included into stdafx.h.
Extracting hardcoded strings from C++ source code
Source files are added and resources are configured and now you are ready to start extracting hard-coded strings.
Select strings in table that you want to extract and click Extract in context menu. Extract strings options dialog will appear. Here you can select destination resource file and configure replace template. The following predefined macro are available.
$(ID) – name of string identifier in resource file. It is equal to $(NUM_ID) if you selected not to create header file.
$(NUM_ID) – numeric resource identifier
$(SRC_STR) – value of hardcoded string (without quotes, brakes, and etc.)
$(SRC_TEXT) – whole string that is replaced with quotes, brakes and modifiers. For example: _T(“Hello World!”)
If you don’t want to extract some string you can select Skip in context menu. The choice will be saved and the string will have Skip status when you decide to extract new strings the next time.
If you want to use the same resource string for several items you can select Use Existing Resource String in context menu.
If you changed source code, you can update files in Extractor project using Scan command from Project menu.
There are several replace templates defined in ResString.h:
- _LS loads string as TCHAR const *.
- _LSW loads string as wchar_t const *
- _LSA loads string as char const *
String is loaded just once at first call.
Adapting source code to use extracted resources
Lingobit Extractor does the most boring and tedious job to extract hard-coded strings. But you need to do several things manually.
- Include new resource file (Sample.RC2) into your main resource file (Sample.RC)
- If you are using build-in replace template _LS, you need to include ResString.h in modified files.
- If you selected to create header file (Sample.h) for extracted resources, you need to include it in in files where new resource ids are used.
Usually it is easier to include ResString.h and Sample.h into precompiled header (stdafx.h).
|